Mengukur Kadar Karbondioksida Dengan Sensor CCS811 dan Mikrokontroler ESP32
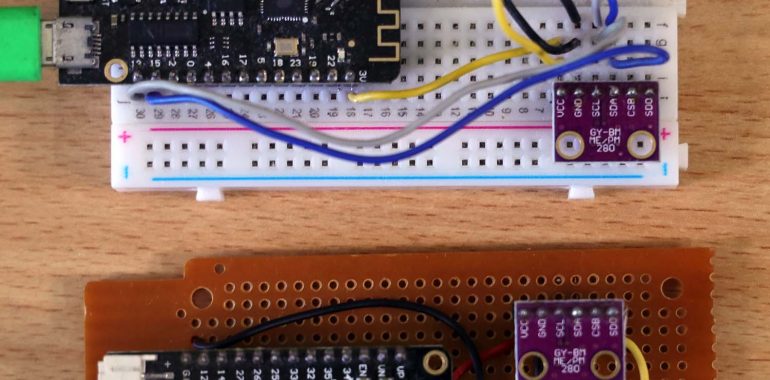
Mengukur Kadar Karbondioksida Dengan Sensor CCS811 dan Mikrokontroler ESP32
Pada percobaan ini dilakukan pengukuran kadar gas CO2 (karbon dioksida) di udara dengan sensor CCS811.
Mikrokontroler yang digunakan adalah modul ESP32 Lolin32 Lite
Modul CJMCU-811 tidak dilengkapi sensor temperatur & kelembaban, sehingga perlu tambahan sensor BME280.
Kompensasi temperatur dan kelembaban untuk sensor CCS811 menggunakan sensor BME280
Berikut ini foto perangkat keras yang digunakan
Berikut ini rangkaian sistem mikroprosesornya
Berikut ini source code program yang dipakai (dengan Arduino)
// https://bitbucket.org/christandlg/bmx280mi/src/master/examples/BMx280_I2C/BMx280_I2C.ino
// BMx280_I2C.ino
//
// shows how to use the BMP280 / BMx280 library with the sensor connected using I2C.
//
// Copyright (c) 2018 Gregor Christandl
//
// connect the AS3935 to the Arduino like this:
//
// Arduino - BMP280 / BME280
// 3.3V ---- VCC
// GND ----- GND
// SDA ----- SDA
// SCL ----- SCL
// some BMP280/BME280 modules break out the CSB and SDO pins as well:
// 5V ------ CSB (enables the I2C interface)
// GND ----- SDO (I2C Address 0x76)
// 5V ------ SDO (I2C Address 0x77)
// other pins can be left unconnected.
// https://github.com/adafruit/Adafruit_CCS811
// https://github.com/adafruit/Adafruit_CCS811/blob/master/examples/CCS811_test/CCS811_test.ino
#include "Adafruit_CCS811.h"
Adafruit_CCS811 ccs;
#include <Arduino.h>
#include <Wire.h>
#define I2C_SDA 15
#define I2C_SCL 13
#include <BMx280I2C.h>
#define I2C_ADDRESS 0x76
//create a BMx280I2C object using the I2C interface with I2C Address 0x76
BMx280I2C bmx280(I2C_ADDRESS);
void setup() {
// put your setup code here, to run once:
Serial.begin(9600);
delay(1000);
//wait for serial connection to open (only necessary on some boards)
while (!Serial);
// Wire.begin();
Wire.begin(I2C_SDA, I2C_SCL);
I2C_Scan() ; // just for verifying
// check CCS811
Serial.println("CCS811 test");
if (!ccs.begin()) {
Serial.println("Failed to start sensor! Please check your wiring.");
while (1);
} else {
Serial.println("CCS811 ready");
}
//begin() checks the Interface, reads the sensor ID (to differentiate between BMP280 and BME280)
//and reads compensation parameters.
if (!bmx280.begin())
{
Serial.println("begin() failed. check your BMx280 Interface and I2C Address.");
while (1);
}
if (bmx280.isBME280())
Serial.println("sensor is a BME280");
else
Serial.println("sensor is a BMP280");
//reset sensor to default parameters.
bmx280.resetToDefaults();
//by default sensing is disabled and must be enabled by setting a non-zero
//oversampling setting.
//set an oversampling setting for pressure and temperature measurements.
bmx280.writeOversamplingPressure(BMx280MI::OSRS_P_x16);
bmx280.writeOversamplingTemperature(BMx280MI::OSRS_T_x16);
//if sensor is a BME280, set an oversampling setting for humidity measurements.
if (bmx280.isBME280())
bmx280.writeOversamplingHumidity(BMx280MI::OSRS_H_x16);
}
void loop() {
float temperature = 0;
float pressure = 0;
float humidity = 60; // default humidity
float co2 = 0;
float tvoc = 0;
delay(1000);
//start a measurement
if (!bmx280.measure())
{
Serial.println("could not start measurement, is a measurement already running?");
return;
}
//wait for the measurement to finish
do
{
delay(100);
} while (!bmx280.hasValue());
// Serial.print("Pressure: "); Serial.println(bmx280.getPressure());
// Serial.print("Pressure (64 bit): "); Serial.println(bmx280.getPressure64());
// Serial.print("Temperature: "); Serial.println(bmx280.getTemperature());
pressure = bmx280.getPressure();
temperature = bmx280.getTemperature();
//important: measurement data is read from the sensor in function hasValue() only.
//make sure to call get*() functions only after hasValue() has returned true.
if (bmx280.isBME280())
{
// Serial.print("Humidity: ");
// Serial.println(bmx280.getHumidity());
humidity = bmx280.getHumidity();
}
ccs.setEnvironmentalData( humidity, temperature );
if (ccs.available()) {
if (!ccs.readData()) {
// Serial.print("CO2: ");
//Serial.print(ccs.geteCO2());
//Serial.print("ppm, TVOC: ");
//Serial.println(ccs.getTVOC());
co2 = ccs.geteCO2();
tvoc = ccs.getTVOC();
}
}
Serial.print("Temp\t");
Serial.print(temperature);
Serial.print("\t");
Serial.print("Humidity\t");
Serial.print(humidity);
Serial.print("\t");
Serial.print("Pressure\t");
Serial.print(pressure);
Serial.print("\t");
Serial.print("CO2\t");
Serial.print(co2);
Serial.print("\t");
Serial.print("TVOC\t");
Serial.print(tvoc);
Serial.println("");
}
void I2C_Scan() {
byte error, address;
int nDevices;
Serial.println("I2C Scanning...");
nDevices = 0;
for (address = 1; address < 127; address++ ) {
Wire.beginTransmission(address);
error = Wire.endTransmission();
if (error == 0) {
Serial.print("I2C device found at address 0x");
if (address < 16) {
Serial.print("0");
}
Serial.println(address, HEX);
nDevices++;
}
else if (error == 4) {
Serial.print("Unknow error at address 0x");
if (address < 16) {
Serial.print("0");
}
Serial.println(address, HEX);
}
}
if (nDevices == 0) {
Serial.println("No I2C devices found\n");
}
else {
Serial.println("done\n");
}
}
Berikut ini software versi lain
// pengukuran CO2, tanpa wifi
// https://bitbucket.org/christandlg/bmx280mi/src/master/examples/BMx280_I2C/BMx280_I2C.ino
// BMx280_I2C.ino
//
// shows how to use the BMP280 / BMx280 library with the sensor connected using I2C.
//
// Copyright (c) 2018 Gregor Christandl
//
// connect the AS3935 to the Arduino like this:
//
// Arduino - BMP280 / BME280
// 3.3V ---- VCC
// GND ----- GND
// SDA ----- SDA
// SCL ----- SCL
// some BMP280/BME280 modules break out the CSB and SDO pins as well:
// 5V ------ CSB (enables the I2C interface)
// GND ----- SDO (I2C Address 0x76)
// 5V ------ SDO (I2C Address 0x77)
// other pins can be left unconnected.
// https://github.com/adafruit/Adafruit_CCS811
// https://github.com/adafruit/Adafruit_CCS811/blob/master/examples/CCS811_test/CCS811_test.ino
#include "Adafruit_CCS811.h"
Adafruit_CCS811 ccs;
#include <Arduino.h>
#include <Wire.h>
#include <BMx280I2C.h>
#include "ClosedCube_HDC1080.h"
#define I2C_SDA 15
#define I2C_SCL 13
#define I2C_ADDRESS 0x76
#define LED_ONBOARD 22
const char *ssid = "First";
const char *password = "satu2345";
//create a BMx280I2C object using the I2C interface with I2C Address 0x76
BMx280I2C bmx280(I2C_ADDRESS);
ClosedCube_HDC1080 hdc1080;
char mac_str[20];
byte mac_byte[6];
void setup() {
pinMode(LED_ONBOARD, OUTPUT);
// put your setup code here, to run once:
Serial.begin(9600);
delay(1000);
//wait for serial connection to open (only necessary on some boards)
while (!Serial);
// Wire.begin();
Wire.begin(I2C_SDA, I2C_SCL);
I2C_Scan() ; // just for verifying
// check CCS811
Serial.println("CCS811 test");
if (!ccs.begin()) {
Serial.println("Failed to start sensor! Please check your wiring.");
while (1);
} else {
Serial.println("CCS811 ready");
}
SetupBME280();
SetupHDC1080();
}
void SetupBME280() {
//begin() checks the Interface, reads the sensor ID (to differentiate between BMP280 and BME280)
//and reads compensation parameters.
if (!bmx280.begin())
{
Serial.println("begin() failed. check your BMx280 Interface and I2C Address.");
while (1);
}
if (bmx280.isBME280())
Serial.println("sensor is a BME280");
else
Serial.println("sensor is a BMP280");
//reset sensor to default parameters.
bmx280.resetToDefaults();
//by default sensing is disabled and must be enabled by setting a non-zero
//oversampling setting.
//set an oversampling setting for pressure and temperature measurements.
bmx280.writeOversamplingPressure(BMx280MI::OSRS_P_x16);
bmx280.writeOversamplingTemperature(BMx280MI::OSRS_T_x16);
//if sensor is a BME280, set an oversampling setting for humidity measurements.
if (bmx280.isBME280())
bmx280.writeOversamplingHumidity(BMx280MI::OSRS_H_x16);
}
void SetupHDC1080() {
hdc1080.begin(0x40);
Serial.println("HDC1080");
Serial.print("Manufacturer ID=0x");
Serial.println(hdc1080.readManufacturerId(), HEX); // 0x5449 ID of Texas Instruments
Serial.print("Device ID=0x");
Serial.println(hdc1080.readDeviceId(), HEX); // 0x1050 ID of the device
hdc1080.setResolution(HDC1080_RESOLUTION_11BIT, HDC1080_RESOLUTION_11BIT);
}
void loop() {
float temperature = 0;
float pressure = 0;
float humidity = 60; // default humidity
float co2 = 0;
float tvoc = 0;
if (1) {
//start a measurement
if (!bmx280.measure())
{
Serial.println("could not start measurement, is a measurement already running?");
}
do
{
delay(100);
} while (!bmx280.hasValue());
pressure = bmx280.getPressure();
temperature = bmx280.getTemperature();
//important: measurement data is read from the sensor in function hasValue() only.
//make sure to call get*() functions only after hasValue() has returned true.
if (bmx280.isBME280())
{
humidity = bmx280.getHumidity();
}
}
ccs.setEnvironmentalData( humidity, temperature ); // kompensasi humidity & temperature
if (ccs.available()) {
if (!ccs.readData()) {
// Serial.print("CO2: ");
//Serial.print(ccs.geteCO2());
//Serial.print("ppm, TVOC: ");
//Serial.println(ccs.getTVOC());
co2 = ccs.geteCO2();
tvoc = ccs.getTVOC();
}
}
Serial.print("Temp\t");
Serial.print(temperature);
Serial.print("\t");
Serial.print("Humidity\t");
Serial.print(humidity);
Serial.print("\t");
Serial.print("Pressure\t");
Serial.print(pressure);
Serial.print("\t");
Serial.print("CO2\t");
Serial.print(co2);
Serial.print("\t");
Serial.print("TVOC\t");
Serial.print(tvoc);
Serial.println("");
delay(500);
digitalWrite(LED_ONBOARD, HIGH);
delay(500);
digitalWrite(LED_ONBOARD, LOW);
}
void I2C_Scan() {
byte error, address;
int nDevices;
Serial.println("I2C Scanning...");
nDevices = 0;
for (address = 1; address < 127; address++ ) {
Wire.beginTransmission(address);
error = Wire.endTransmission();
if (error == 0) {
Serial.print("I2C device found at address 0x");
if (address < 16) {
Serial.print("0");
}
Serial.println(address, HEX);
nDevices++;
}
else if (error == 4) {
Serial.print("Unknow error at address 0x");
if (address < 16) {
Serial.print("0");
}
Serial.println(address, HEX);
}
}
if (nDevices == 0) {
Serial.println("No I2C devices found\n");
}
else {
Serial.println("done\n");
}
}
Berikut ini contoh tampilan outputnya
13:08:18.861 -> I2C Scanning...
13:08:18.861 -> I2C device found at address 0x5A
13:08:18.896 -> I2C device found at address 0x76
13:08:18.931 -> done
13:08:18.931 ->
13:08:18.931 -> CCS811 test
13:08:19.101 -> CCS811 ready
13:08:19.101 -> sensor is a BME280
13:08:19.135 -> HDC1080
13:08:19.135 -> Manufacturer ID=0xFFFF
13:08:19.135 -> Device ID=0xFFFF
13:08:19.239 -> Temp 30.83 Humidity 50.10 Pressure 87156.00 CO2 0.00 TVOC 0.00
13:08:20.368 -> Temp 30.83 Humidity 50.29 Pressure 91715.00 CO2 0.00 TVOC 0.00
13:08:21.464 -> Temp 30.84 Humidity 50.37 Pressure 91714.00 CO2 0.00 TVOC 0.00
Referensi
- Contoh program BME280 https://bitbucket.org/christandlg/bmx280mi/src/master/examples/BMx280_I2C/BMx280_I2C.ino
- Contoh program CCS811 https://github.com/adafruit/Adafruit_CCS811/blob/master/examples/CCS811_test/CCS811_test.ino